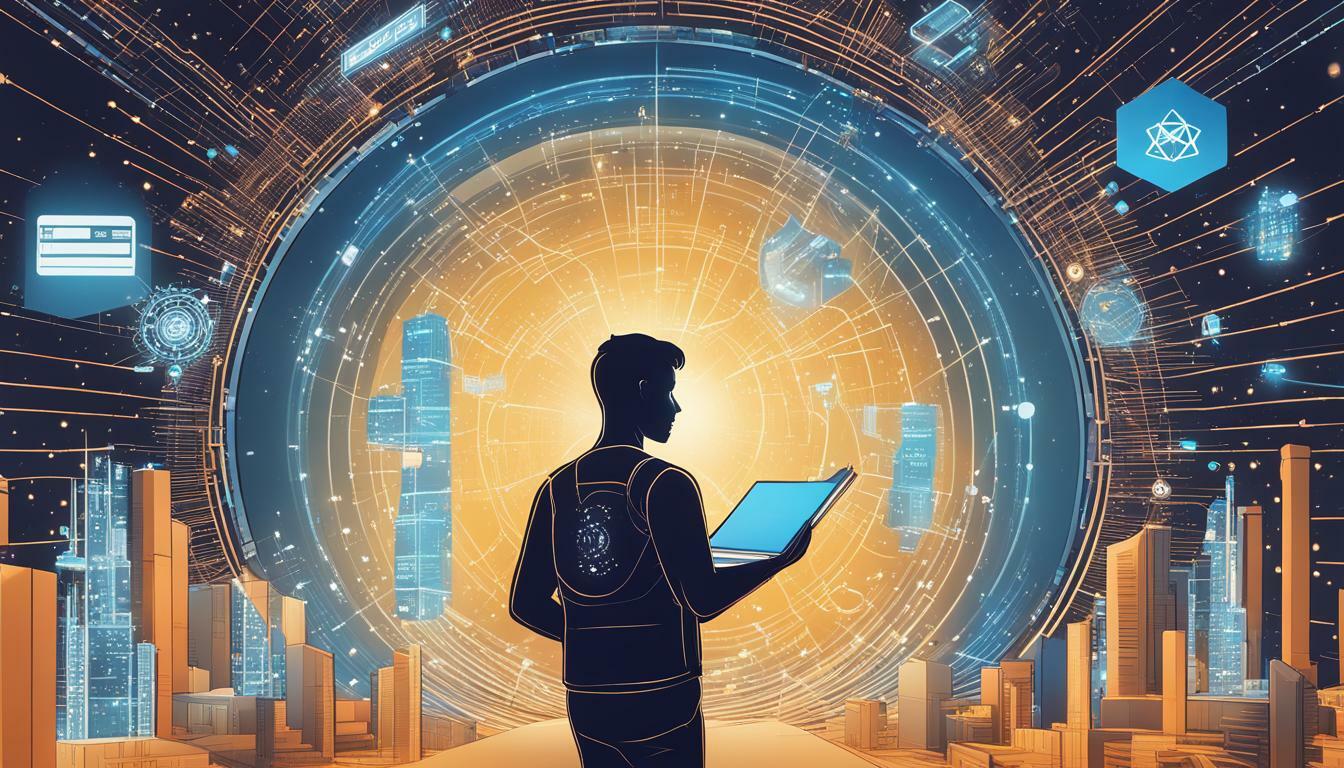
Welcome to our comprehensive guide on API development using .NET Core and C#! APIs have become an essential part of modern programming by enabling applications to communicate and share data with ease. In this article, we will introduce you to the benefits of using .NET Core and C# for API development and provide you with a step-by-step guide for building robust and efficient APIs.
If you are a developer looking to enhance your API development skills, you’ve come to the right place. Let’s get started!
Key Takeaways:
- API development using .NET Core and C# is a powerful way to build modern applications.
- .NET Core provides a cross-platform development environment with improved performance and a rich ecosystem of libraries and tools.
- C# is a versatile programming language with advanced features that can be utilized to build robust and efficient APIs.
- This article will provide you with a step-by-step guide on how to build, test, debug, and secure APIs using .NET Core and C#.
Understanding API Development
If you’re new to API development, it might seem like an intimidating topic. However, with the right guidance, anyone can learn how to develop APIs using .NET Core and C#. In this section, we’ll go over the core concepts and principles of API development, providing you with the necessary foundation to start building your own APIs.
What is an API?
API stands for Application Programming Interface. It’s essentially a set of protocols, routines, and tools for building software applications. APIs allow different software applications to communicate with each other, enabling them to share data and functionality. In other words, APIs make it easy for developers to create software that can interact with other software.
RESTful APIs
A RESTful API is an architectural style for building APIs. It stands for Representational State Transfer and is designed to be simple, lightweight, and scalable. RESTful APIs use HTTP methods such as GET, POST, PUT, and DELETE to interact with resources, which can be represented in different formats such as JSON or XML.
HTTP Methods
HTTP methods are the main means of interaction with RESTful APIs. Here are some common HTTP methods:
- GET – retrieves a resource
- POST – creates a new resource
- PUT – updates an existing resource
- DELETE – deletes a resource
Request/Response Handling
When a client makes a request to an API, the API must be able to handle the request and send back a response. Request handling involves parsing the incoming request, processing it, and generating a response. Response handling involves generating a response, serializing it, and sending it back to the client.
Data Serialization
Data serialization is the process of converting objects or data structures into a format that can be easily transmitted over a network or stored in a file. Common serialization formats include JSON and XML. Serialization is a key aspect of API development, as it allows data to be easily consumed and processed by different applications.
Now that we’ve covered the core concepts of API development, you’re ready to move on to the next section where we’ll introduce you to .NET Core.
Introducing .NET Core
If you’re looking to develop APIs, .NET Core is a powerful and flexible framework that can help you accomplish your goals. Developed by Microsoft, .NET Core is an open-source, cross-platform framework that supports various programming languages, including C#. With .NET Core, you can build APIs that are fast, secure, and scalable.
One of the main advantages of using .NET Core for API development is the performance improvements it offers. .NET Core is designed to be lightweight, allowing your APIs to run faster and consume fewer resources. Additionally, .NET Core offers cross-platform compatibility, meaning you can develop and deploy your APIs on a wide range of operating systems, including Windows, Linux, and macOS.
Another benefit of using .NET Core is the rich ecosystem of libraries and tools that are available. With .NET Core, you have access to a vast selection of libraries for data manipulation, networking, security, and more. You can also use popular tools like Visual Studio, Visual Studio Code, and Azure DevOps to streamline your development process.
Mastering C# for API Development
As mentioned earlier, C# plays a crucial role in the development of APIs using .NET Core. With its powerful features and capabilities, C# in action can help you build robust and efficient APIs that can handle complex business logic and data operations.
Object-oriented programming (OOP) is one of the key features of C# that makes it ideal for API development. Using classes and objects, you can structure your code in a modular and reusable way, making it easier to maintain and scale.
LINQ (Language-Integrated Query) is another powerful feature of C# that can simplify data access and manipulation in your APIs. With LINQ, you can write queries in a simple and intuitive syntax, allowing you to retrieve, filter, and transform data from various sources such as databases and JSON files.
Asynchronous programming using async/await is also an essential skill for API development in C#. By using async/await, you can write code that can handle multiple requests and operations concurrently without blocking the main thread.
Exception handling is another important aspect of API development in C#. By properly handling exceptions, you can ensure that your APIs are resilient and able to recover from errors gracefully, providing a better user experience.
With these and other features of C# in action, you can master the art of API development using C# and create high-quality APIs that meet the needs of your users and business.
Building APIs with .NET Core and C#
Now that you have a solid understanding of API development and the benefits of using .NET Core and C#, it’s time to start building your own APIs. Follow these steps to get started:
Step 1: Setting up a development environment
The first step to building APIs with .NET Core and C# is setting up your development environment. You’ll need to download and install the .NET Core SDK, which includes the necessary tools and libraries to build and run .NET Core applications. You can do this from the official .NET Core website.
Step 2: Creating controllers and routes
Once you have your development environment set up, you can start creating controllers and routes. Controllers are responsible for handling incoming HTTP requests, while routes define the URL patterns that map to specific controller actions. To create a new controller in .NET Core, you can use the scaffolding tools provided by the CLI or Visual Studio.
Step 3: Handling authentication and authorization
APIs often need to handle authentication and authorization to ensure that only authorized users can access certain resources. You can use the built-in authentication and authorization middleware provided by .NET Core to implement these features in your APIs. This includes support for OAuth, OpenID Connect, and JWT tokens.
Step 4: Implementing data persistence using Entity Framework Core
Finally, you’ll need to implement data persistence in your APIs. Entity Framework Core is a powerful ORM (Object-Relational Mapping) framework that allows you to work with databases using C# code. With Entity Framework Core, you can define your database schema using C# classes and LINQ queries, making it easy to work with data in your APIs.
By following these steps, you’ll be well on your way to building powerful APIs with .NET Core and C#. With their rich ecosystem of libraries and tools, and support for cross-platform development, you’ll be able to create APIs that are both robust and efficient.
Testing and Debugging APIs
Testing and debugging are integral parts of API development using .NET Core and C#. They help ensure that the APIs are reliable and perform as expected. In this section, we will discuss various testing techniques and tools available for API development using .NET Core and C#.
Unit Testing
Unit testing is a testing technique in which individual units or components of software are tested in isolation from the rest of the system. In the context of API development, unit testing involves testing individual API endpoints or methods.
In .NET Core, the XUnit and NUnit testing frameworks are popular choices for unit testing. These frameworks provide a comprehensive set of tools and APIs for writing and executing unit tests. They also support test-driven development (TDD), which involves writing tests before writing the actual code.
Integration Testing
Integration testing involves testing the interactions between different components or modules of the system. In the context of API development, integration testing involves testing the interactions between different API endpoints or methods.
In .NET Core, the Microsoft.AspNetCore.Mvc.Testing package provides a set of tools and APIs for integration testing. It allows you to simulate HTTP requests and responses and test the behavior of your API under different conditions.
Debugging Tools
Debugging is the process of finding and fixing errors or bugs in software. In the context of API development, debugging involves identifying and resolving issues in the API code.
In .NET Core, the Visual Studio IDE provides a rich set of debugging tools for API development. These tools allow you to step through code, inspect variables, and track the flow of execution. They also provide diagnostic information, such as stack traces and error messages, to help you identify and resolve issues.
Securing APIs
As more and more businesses rely on APIs for their day-to-day operations, ensuring the security of these APIs has become a critical concern. In API development with .NET Core and C#, security is an essential component that needs to be carefully considered.
.NET Core API development offers several built-in mechanisms to secure your APIs. One of the most common security measures is authentication, which verifies the identity of the user making the request. This can be achieved through various mechanisms such as token-based authentication, OAuth, or OpenID Connect.
Authorization is another important aspect of API security. Ensuring that only authorized users can access sensitive data or perform critical operations is crucial. In .NET Core API development, authorization can be achieved through role-based access control (RBAC), policies, or custom authorization filters.
Another area where security needs to be carefully considered is in protecting sensitive data in transit and at rest. .NET Core API development provides several encryption and decryption mechanisms that can be used to safeguard sensitive data. These include the use of SSL/TLS certificates, data encryption at rest, and secure storage of secrets and keys.
Finally, it is essential to protect your APIs against common security vulnerabilities such as cross-site scripting (XSS), SQL injection, or cross-site request forgery (CSRF). .NET Core API development provides various built-in measures to prevent these vulnerabilities, such as request validation, input parameter validation, and output encoding.
In summary, securing your APIs is a crucial aspect of API development with .NET Core and C#. By carefully considering authentication, authorization, data protection, and vulnerability prevention, you can ensure that your APIs are secure and reliable.
Conclusion
API development using .NET Core and C# is a powerful and flexible way to build modern, scalable, and secure applications. In this article, we have explored the fundamentals of API development and how .NET Core and C# can be utilized to create high-quality APIs that meet today’s complex business requirements.
We have discussed various aspects of API development, including understanding the basic concepts and principles, getting familiar with .NET Core and C#, building APIs from scratch, testing and debugging APIs, and securing APIs. We hope that this comprehensive guide has given you a solid foundation to start building your own APIs using these powerful technologies.
Final Thoughts
As you continue to explore and improve your API development skills, we encourage you to leverage the vast resources available online, including the official documentation and community forums for .NET Core and C#. By using these resources, you can stay up-to-date with the latest advancements and best practices in API development, and hone your skills to become a proficient API developer.
We hope this article has been a valuable resource for you, and wish you the best of luck in your journey towards becoming a skilled API developer with .NET Core and C#.
FAQ
Q: What is API development?
A: API development refers to the process of creating application programming interfaces, which allow different software applications to communicate and interact with each other. APIs define the methods and protocols that enable data exchange between applications, enabling developers to build new features or integrate existing systems.
Q: Why is API development important?
A: API development is important because it enables seamless integration and collaboration between different software applications. APIs simplify the process of sharing data and functionality between systems, making it easier for developers to create new applications, build on top of existing platforms, and enhance user experiences.
Q: What is .NET Core?
A: .NET Core is a cross-platform, open-source framework developed by Microsoft. It provides a runtime and libraries for building applications for various platforms, including Windows, macOS, and Linux. .NET Core offers performance improvements, a modular architecture, and a rich ecosystem of libraries and tools for application development.
Q: What is C#?
A: C# is a modern, object-oriented programming language developed by Microsoft. It is widely used to build a wide range of applications, including web and desktop applications, games, and mobile apps. C# is known for its simplicity, readability, and robustness, making it a popular choice for API development.
Q: How do I build APIs using .NET Core and C#?
A: To build APIs using .NET Core and C#, you can follow a step-by-step process that includes setting up a development environment, creating controllers and routes, handling authentication and authorization, and implementing data persistence using Entity Framework Core. Detailed tutorials and documentation are available to guide you through the process.
Q: How can I test and debug APIs?
A: Testing and debugging APIs is essential to ensure their quality and reliability. Various techniques and tools, such as unit testing frameworks, integration testing frameworks, and debugging tools, are available for API development using .NET Core and C#. These tools help identify and fix issues in the code, ensuring the API functions as intended.
Q: How can I secure my APIs?
A: Securing APIs is a crucial aspect of development. Best practices for API security include implementing authentication and authorization mechanisms, securing sensitive data using encryption and secure protocols, and protecting against common security vulnerabilities, such as SQL injection and cross-site scripting. Proper security measures help protect user data and maintain the integrity of the API.